In this part of the PHP GTK programming tutorial, we will create a Nibbles game clone.
Nibbles is an older classic video game. It was first created in late 70s. Later it was brought to PCs. In this game the player controls a snake. The objective is to eat as many apples as possible. Each time the snake eats an apple, its body grows. The snake must avoid the walls and its own body.
The code is split into two files. board.php and nibbles.php.
The
If the game is over, we call the
In the
In the
The
In the
Nibbles is an older classic video game. It was first created in late 70s. Later it was brought to PCs. In this game the player controls a snake. The objective is to eat as many apples as possible. Each time the snake eats an apple, its body grows. The snake must avoid the walls and its own body.
Development
The size of each of the joints of a snake is 10px. The snake is controlled with the cursor keys. Initially, the snake has three joints. The game starts immediately. When the game is finished, we display "Game Over" message in the center of the window.The code is split into two files. board.php and nibbles.php.
<?php // board.php define("WIDTH", 300); define("HEIGHT", 270); define("DOT_SIZE", 10); define("ALL_DOTS", WIDTH * HEIGHT / (DOT_SIZE * DOT_SIZE)); define("RAND_POS", 26); class Board extends GtkDrawingArea { public function __construct() { parent::__construct(); $this->modify_bg(Gtk::STATE_NORMAL, new GdkColor(6400, 6400, 6440)); $this->connect('expose_event', array($this, 'on_expose')); $this->init_game(); } public function init_game() { $this->x = array_fill(0, ALL_DOTS, 0); $this->y = array_fill(0, ALL_DOTS, 0); $this->left = false; $this->right = true; $this->up = false; $this->down = false; $this->inGame = true; $this->dots = 3; for ($i=0; $i<=$this->dots; $i++) { $this->x[$i] = 50 - $i * 10; $this->y[$i] = 50; } try { $this->dot = CairoImageSurface::createFromPng("dot.png"); $this->head = CairoImageSurface::createFromPng("head.png"); $this->apple = CairoImageSurface::createFromPng("apple.png"); } catch( Exception $e) { echo $e->getMessage(); echo "cannot load images"; exit; } $this->locate_apple(); $this->set_can_focus(true); Gtk::timeout_add(100, array($this, 'on_timer')); } public function on_timer() { if ($this->inGame) { $this->check_apple(); $this->check_collision(); $this->move(); $this->queue_draw(); return true; } else { return false; } } public function on_expose() { $cr = $this->window->cairo_create(); if ($this->inGame) { $this->draw_objects($cr); } else { $this->game_over($cr); } } public function draw_objects($cr) { $cr->SetSourceRgb(0, 0, 0); $cr->paint(); $cr->setSourceSurface($this->apple, $this->apple_x, $this->apple_y); $cr->paint(); for ($z=0; $z<=$this->dots; $z++) { if ($z == 0) { $cr->setSourceSurface($this->head, $this->x[$z], $this->y[$z]); $cr->paint(); } else { $cr->setSourceSurface($this->dot, $this->x[$z], $this->y[$z]); $cr->paint(); } } } public function game_over($cr) { $c_x = $this->get_allocation()->width/2; $c_y = $this->get_allocation()->height/2; $cr->SetFontSize(15); $cr->SetSourceRgb(65535, 65535, 65535); $te = $cr->TextExtents("Game Over"); $cr->MoveTo($c_x - $te['width']/2, $c_y); $cr->ShowText("Game Over"); } public function check_apple() { if ($this->x[0] == $this->apple_x and $this->y[0] == $this->apple_y) { $this->dots = $this->dots + 1; $this->locate_apple(); } } public function move() { $z = $this->dots; while ($z > 0) { $this->x[$z] = $this->x[($z - 1)]; $this->y[$z] = $this->y[($z - 1)]; $z--; } if ($this->left) { $this->x[0] -= DOT_SIZE; } if ($this->right) { $this->x[0] += DOT_SIZE; } if ($this->up) { $this->y[0] -= DOT_SIZE; } if ($this->down) { $this->y[0] += DOT_SIZE; } } public function check_collision() { $z = $this->dots; while ($z > 0) { if ($z > 4 and $this->x[0] == $this->x[$z] and $this->y[0] == $this->y[$z]) { $this->inGame = false; } $z--; } if ($this->y[0] > HEIGHT - DOT_SIZE) { $this->inGame = false; } if ($this->y[0] < 0) { $this->inGame = false; } if ($this->x[0] > WIDTH - DOT_SIZE) { $this->inGame = false; } if ($this->x[0] < 0) { $this->inGame = false; } } public function locate_apple() { $r = rand(0, RAND_POS); $this->apple_x = $r * DOT_SIZE; $r = rand(0, RAND_POS); $this->apple_y = $r * DOT_SIZE; } public function on_key_down($event) { $key = $event->keyval; if ($key == Gdk::KEY_Left and !$this->right) { $this->left = true; $this->up = false; $this->down = false; } if ($key == Gdk::KEY_Right and !$this->left) { $this->right = true; $this->up = false; $this->down = false; } if ($key == Gdk::KEY_Up and !$this->down) { $this->up = true; $this->right = false; $this->left = false; } if ($key == Gdk::KEY_Down and !$this->up) { $this->down = true; $this->right = false; $this->left = false; } } } ?>This is board.php file.
define("WIDTH", 300); define("HEIGHT", 270); define("DOT_SIZE", 10); define("ALL_DOTS", WIDTH * HEIGHT / (DOT_SIZE * DOT_SIZE)); define("RAND_POS", 26);The WIDTH and HEIGHT constants determine the size of the Board. The DOT_SIZE is the size of the apple and the dot of the snake. The ALL_DOTS constant defines the maximum number of possible dots on the Board. The RAND_POS constant is used to calculate a random position of an apple.
$this->x = array_fill(0, ALL_DOTS, 0); $this->y = array_fill(0, ALL_DOTS, 0);These two arrays store x, y coordinates of all possible joints of a snake.
The
init_game()
method initializes variables, loads
images and starts a timeout function.
if ($this->inGame) { $this->check_apple(); $this->check_collision(); $this->move(); $this->queue_draw(); return true; } else { return false; }Every 140 ms, the
on_timer()
method is called. If
we are in the game, we call three methods, that build the logic of the game.
The queue_draw()
method forces the widget to be redrawn. This
will reflect the changes of the game on the board.
Otherwise we return false
, which stops the timer event.
$cr = $this->window->cairo_create(); if ($this->inGame) { $this->draw_objects($cr); } else { $this->game_over($cr); }Inside the
on_expose()
method, we check the $this->inGame
variable. If it is true, we draw our objects. The apple and the snake joints.
Otherwise we display "Game over" text.
public function draw_objects($cr) { $cr->SetSourceRgb(0, 0, 0); $cr->paint(); $cr->setSourceSurface($this->apple, $this->apple_x, $this->apple_y); $cr->paint(); for ($z=0; $z<=$this->dots; $z++) { if ($z == 0) { $cr->setSourceSurface($this->head, $this->x[$z], $this->y[$z]); $cr->paint(); } else { $cr->setSourceSurface($this->dot, $this->x[$z], $this->y[$z]); $cr->paint(); } } }The
draw_objects()
method draws the apple and the joints of the
snake. The first joint of a snake is its head, which is represented by a red circle.
If the game is over, we call the
game_over()
method. This method shows "Game Over" in the center of the window.
$c_x = $this->get_allocation()->width/2; $c_y = $this->get_allocation()->height/2;Here we get the center point of the window.
$cr->SetFontSize(15); $cr->SetSourceRgb(65535, 65535, 65535);We set the font size and color for the text. The background is black so the font will be in white color.
$te = $cr->TextExtents("Game Over");We get the text extents of the string. This is needed in order to position the text in the center of the window.
$cr->MoveTo($c_x - $te['width']/2, $c_y); $cr->ShowText("Game Over");We move to the center and display the text.
public function check_apple() { if ($this->x[0] == $this->apple_x and $this->y[0] == $this->apple_y) { $this->dots = $this->dots + 1; $this->locate_apple(); } }The
check_apple()
method checks, if the snake has hit
the apple object. If so, we add another snake joint and call the
locate_apple()
method, which randomly places a new apple object.
In the
move()
method we have the key algorithm of the game.
To understand it, look at how the snake is moving. You control the head of the snake.
You can change its direction with the cursor keys. The rest of the joints move
one position up the chain. The second joint moves where the first was,
the third joint where the second was etc.
while ($z > 0) { $this->x[$z] = $this->x[($z - 1)]; $this->y[$z] = $this->y[($z - 1)]; $z--; }This code moves the joints up the chain.
if ($this->left) { $this->x[0] -= DOT_SIZE; }Move the head to the left.
In the
check_collision()
method, we determine if the snake
has hit itself or one of the walls.
while ($z > 0) { if ($z > 4 and $this->x[0] == $this->x[$z] and $this->y[0] == $this->y[$z]) { $this->inGame = false; } $z--; }Finish the game, if the snake hits one of its joints with the head.
if ($this->y[0] > HEIGHT - DOT_SIZE) { $this->inGame = false; }Finish the game, if the snake hits the bottom of the Board.
The
locate_apple()
method locates an apple randomly
on the board.
$r = rand(0, RAND_POS);We get a random number from 0 to RAND_POS - 1.
$this->apple_x = $r * DOT_SIZE; ... $this->apple_y = $r * DOT_SIZE;These lines set the x, y coordinates of the apple object.
In the
on_key_down()
method of the Board class, we
determine the keys that were pressed.
if ($key == Gdk::KEY_Left and !$this->right) { $this->left = true; $this->up = false; $this->down = false; }If we hit the left cursor key, we set
$this->left
variable to
true. This variable is used in the move()
method to change coordinates of the snake object. Notice also, that
when the snake is heading to the right, we cannot turn immediately
to the left.
<?php /* ZetCode PHP GTK tutorial In this program, we create a Nibbles game clone. author: Jan Bodnar website: www.zetcode.com last modified: September 2011 */ include 'board.php'; class Example extends GtkWindow { public function __construct() { parent::__construct(); $this->init_ui(); } private function init_ui() { $this->set_title('Nibbles'); $this->connect_simple('destroy', array('gtk', 'main_quit')); $this->board = new Board(); $this->board->connect('key-press-event', array($this, 'on_key_down')); $this->add($this->board); $this->set_default_size(300, 270); $this->set_position(GTK::WIN_POS_CENTER); $this->show_all(); } public function on_key_down($sender, $event) { $key = $event->keyval; $this->board->on_key_down($event); } } new Example(); Gtk::main(); ?>This is the nibbles.php file. In this file, we set up the Nibbles game.
public function on_key_down($sender, $event) { $key = $event->keyval; $this->board->on_key_down($event); }In this class, we catch the key press events. And delegate the processing to the
on_key_down()
method of the board class.
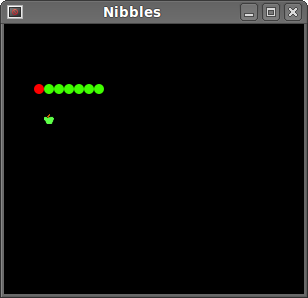
Figure: Nibbles
This was the Nibbles computer game programmed with the GTK library
and the PHP programming language.